Intro #
In this quick start, we are going to build a simple resource-based building system with UE5.0, default third person template, and this plugin. This system contains these features:
- Can choose what to build
- Show the preview item, and move it in front of the character
- Allow player to rotate the preview item
- Check if resources are enough to build that item when trying to build.
- Process resources cost when item is built.
Video walkthrough #
The full process can be found here.
We recommend that you follow this document. Please note that there are no voice or text instructions provided in the accompanying video.
Project setup #
- Create a new project with the Third Person template.
- Activate tiled level plugin, check its version is >= V2.0.0.
- Clean up the default map by removing all existing meshes, and put a simple plane as floor and scale it to [50, 50, 1] (or larger). Set the location of the plane to [0, 0, 0] to match the default start z location of the system. You may set a better looking material for that floor plane.
Create tiled level gametime system #
- Create a blank gameinstance blueprint and set it to your Game Instance Class. (Project Settings -> Maps & Modes)
It seems that you need a custom gameinstance class to let game instance subsystem work correctly!
- Create a blueprint inherited from TiledlevelGametimeSystem. It’s a UGameInstanceSubsystem that you can get access to it everywhere with life span across the whole game play. Check out the Programming Subsystems if you are not familiar with. Rename the blueprint as BP_BuildSystem.
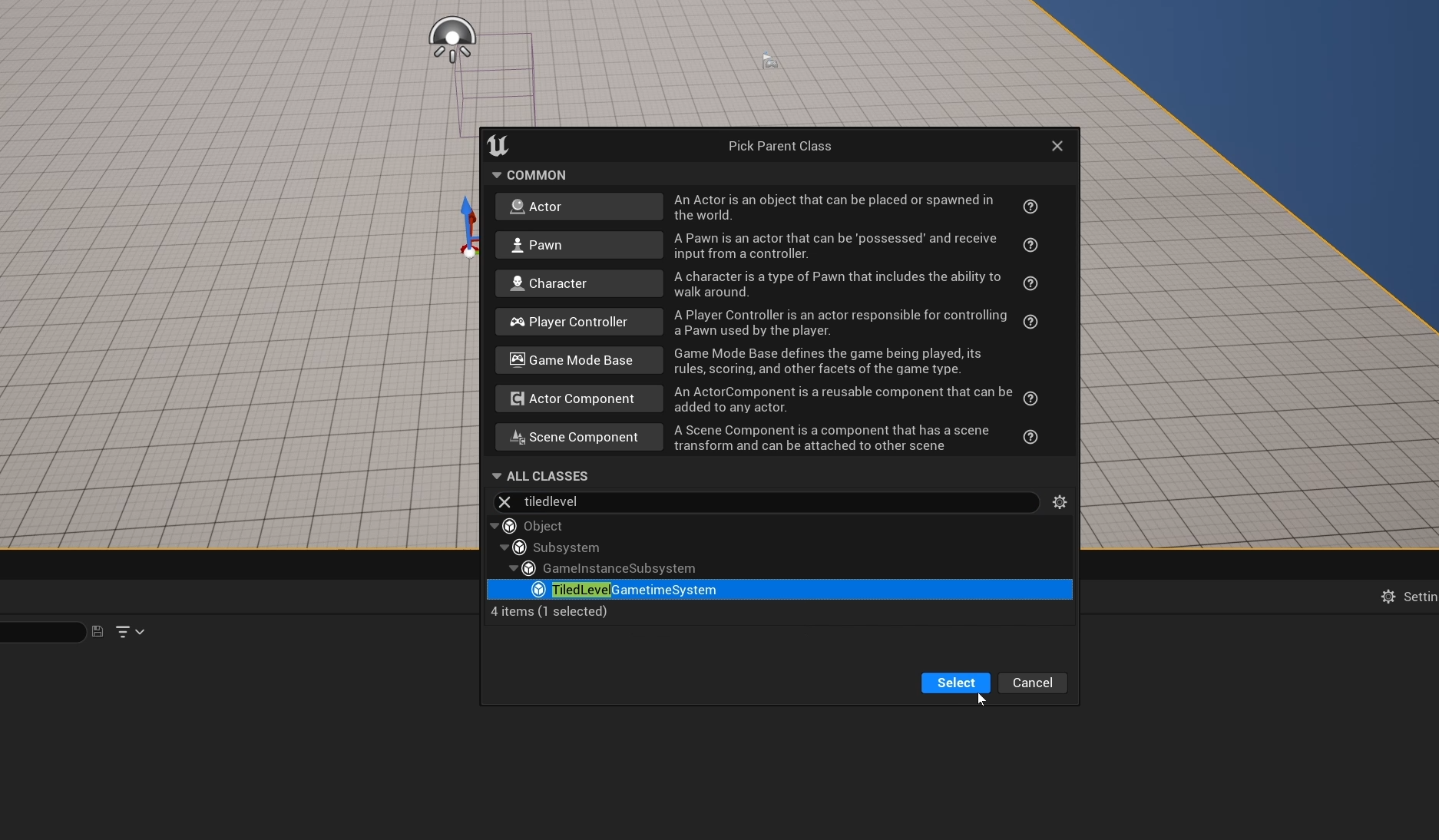
- Inside class default, set tile size as [250, 250, 250], and create new integer variable Wood and Stone as the current resources. Set their default value to 100.
Create item set #
- Create a new item set called TS_House, set tile size to [250, 250, 250], and add a few meshes from HousePack (Plugin/TiledLevel/DemoHouse/Meshes).
Create custom data and link with item set #
- Create a Structure called SHouseItemInfo. Create 4 variables: Name (Text), Description (Text), WoodCost (integer), and StoneCost (integer).
- Create a Data Table called DT_HouseItemInfo with its row structure as SHouseItemInfo. There is no need to edit any content for this data table, just close it.
- Under TS_House set the custom data table to DT_HouseItemInfo and click Initialize Data.
After filling the custom info for each item, the setup at this stage is completed.
Known crash issue: Modify the Structure and go back to its associated opened Item Set will crash the editor for now. You should close the Item Set before modify the Struct.
Finish your gametime system blueprint #
With custom data properly setup, we can continue the rest logic in BP_BuildSystem.
Override two functions: Begin System and CanBuildItem.
- For CanBuildItem create the logic to check whether current resources is enough to build that particular item.
- After Begin System, bind a custom event to OnItemBuilt to add a simple logic to subtract resource cost for the newly built item.
For more information, check out Override Functions.
Bind control #
Open BP_ThirdPersonCharacter and create the following logic.
- Add Begin Play, promote table row names from DT_HouseItemInfo to a private variable AvailableItemIDs.
- Create a custom event ActivatePreviewFromSelectedID.
- Add input event Wheel up and Wheel down to implement how to choose item and activated them. Create a integer variable SelectedItemIndex and follows the graph below.
- Add input event Left Mouse Button to build item.
- Add input event R to rotate preview item.
- Add input event Right Mouse Button to deactivate preview item.
Last, you need to let the preview item move along with the character.
- Add Tick and calculate the forward 250 cm to move the preview item.
Create basic UI #
Create a basic UI to display the current resource utilization, allowing for easy tracking of resource consumption.
- Create an UserWidget called WBP_UI. Place 2 TextBlock in canvas, and bind their GetText function as below.
- Enable this widget in BP_ThirdPersonCharacter.
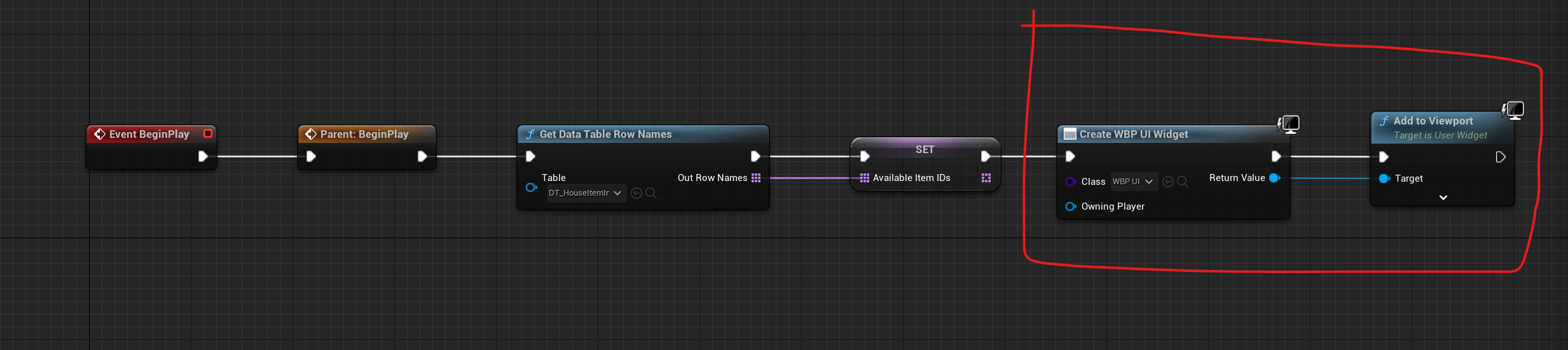
Initialize gametime system #
In level blueprint (or anywhere you like) call Initialize Gametime System and set Startup Item Set as TS_House and set Unbound true.
Done! #
Now hit play and have fun!
What’s next? #
Checkout here for other related topic about gametime system.